App Icon Shortcut
Android Name: Application Icon Shortcut
iOS Name: Home Quick Action
App Icon Shortcuts are easy way to access certain feature(s) inside of your application. It behaves similarly with deeplink. You would actually be able to use the same sub-methods for Icon Shortcuts and deeplink. For more info about deeplink:
Universal LinksSince App Icon Shortcut is a native feature, it has different implementation on Android and iOS. We have to make our changes through AndroidManifest.xml and Info.plist respectively.
This feature only works on Published Application. It will not work on Smartface Emulator. To test it easily, you can use Appcircle Device Preview feature.
Android Implementation of App Icon Shortcut
Android Documentation:
https://developer.android.com/guide/topics/ui/shortcuts
On Android, App Icon Shortcut can be accessed via long pressing the Application Icon.
Most Android devices support up to four(4) different shortcuts at one time. It is not recommended to add more.
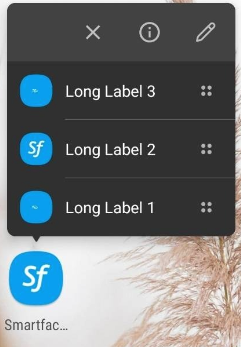
If you are implementing the App Icon Shortcut to a project which is created before version 6.16.8, you need to create following two folders:
- config/Android/shortcuts.xml
- config/Android/strings.xml
We will also need to edit our AndroidManifest.xml
file.
AndroidManifest.xml
Add the code on the 9th line.
<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.myapplication">
<application ... >
<activity>
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
<!-- add this element -->
<meta-data android:name="android.app.shortcuts" android:resource="@xml/shortcuts" />
</activity>
</application>
</manifest>
shortcuts.xml
This file will contain our metadata about shortcuts. It follows the syntax at the Android Documentation below:
https://developer.android.com/guide/topics/ui/shortcuts/creating-shortcuts#attribute-values
Each element contains a static shortcut and relevant intent info of the shortcut. An example:
<shortcuts xmlns:android="http://schemas.android.com/apk/res/android">
<shortcut android:shortcutId="shortcutTest" android:enabled="true" android:icon="@drawable/icon" android:shortcutShortLabel="@string/shortcut_short_label" android:shortcutLongLabel="@string/shortcut_short_label" android:shortcutDisabledMessage="@string/shortcut_short_label">
<intent android:action="android.intent.action.VIEW" android:targetPackage="${PackageName}" android:targetClass="${PackageName}.A">
<!-- This element is important! -->
<extra android:name="AppShortcutType" android:value="AppShortcutType" />
<!-- You can pass data via adding more extra -->
<extra android:name="route" android:value="page1" />
</intent>
<!-- <categories android:name="android.shortcut.conversation" /> -->
<!-- <capability-binding android:key="actions.intent.CREATE_MESSAGE" /> -->
</shortcut>
<shortcut android:shortcutId="shortcutTest" android:enabled="true" android:icon="@drawable/icon" android:shortcutShortLabel="@string/shortcut_short_label" android:shortcutLongLabel="@string/shortcut_short_label" android:shortcutDisabledMessage="@string/shortcut_short_label">
<intent android:action="android.intent.action.VIEW" android:targetPackage="${PackageName}" android:targetClass="${PackageName}.A">
<!-- This element is important! -->
<extra android:name="AppShortcutType" android:value="AppShortcutType" />
<!-- You can pass data via adding more extra -->
<extra android:name="route" android:value="page2" />
</intent>
<!-- <categories android:name="android.shortcut.conversation" /> -->
<!-- <capability-binding android:key="actions.intent.CREATE_MESSAGE" /> -->
</shortcut>
</shortcuts>
The properties:
- android:icon="@drawable/image_name" = images/Android/drawable/image_name
- You can use the image name you used to generate the image through Smartface Image Generator
- @string/ refer to config/Android/strings.xml file.
The name and value on the extra will be sent to the callback Application.onAppShortcutReceived
Application.onAppShortcutReceived = (e: { data: { [key: string]: any } }) => {
console.log(e); //this will log { "route": "page1" } when clicked on the first shortcut
};
For more capabilities, check this document:
https://developer.android.com/guide/topics/ui/shortcuts/creating-shortcuts#inner-elements
strings.xml
In order to define the @string/
values, keep them organized in this file. Example:
<resources>
<string name="shortcut_short_label">Short Label</string>
<string name="shortcut_short_label">Long Label</string>
<string name="shortcut_short_label">Disable Message</string>
</resources>
iOS Implementation of Home Quick Actions
Apple Documentation:
https://developer.apple.com/documentation/uikit/menus_and_shortcuts/add_home_screen_quick_actions
Home Quick Actions are supported on iOS 13 and more.
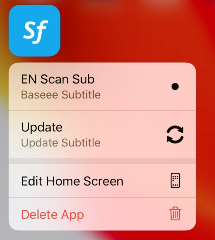
For Images, Apple recommends
104x104
dimension size for 3x. When you are using Smartface Image Generator, it means your original image size should be32x32
.
For iOS, there are no new files needed. Add this key/array entry to your Info.plist:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>UIApplicationShortcutItems</key>
<array>
<dict>
<key>UIApplicationShortcutItemIconFile</key>
<string>Scan</string>
<key>UIApplicationShortcutItemTitle</key>
<string>SCAN_SHORTCUT_TITLE</string>
<key>UIApplicationShortcutItemSubtitle</key>
<string>SCAN_SHORTCUT_SUBTITLE</string>
<key>UIApplicationShortcutItemType</key>
<string>Smartface.Scan</string>
<key>UIApplicationShortcutItemUserInfo</key>
<dict>
<key>route</key>
<string>page1</string>
</dict>
</dict>
<dict>
<key>UIApplicationShortcutItemIconFile</key>
<string>Update</string>
<key>UIApplicationShortcutItemTitle</key>
<string>Update</string>
<key>UIApplicationShortcutItemSubtitle</key>
<string>Update Subtitle</string>
<key>UIApplicationShortcutItemType</key>
<string>Smartface.Update</string>
<key>UIApplicationShortcutItemUserInfo</key>
<dict>
<key>route</key>
<string>page2</string>
</dict>
</dict>
</array>
<!--your rest of plist-->
</dict>
</plist>
The strings should be added to Localization strings file. Example for English:
"Local_DisplayName" = "ENDisplay";
"SCAN_SHORTCUT_TITLE" = "EN Scan";
"SCAN_SHORTCUT_SUBTITLE" = "EN Scan Sub"
More info about Localization:
LocalizationThe name and value on the extra will be sent to the callback Application.onAppShortcutReceived
Application.onAppShortcutReceived = (e: { data: { [key: string]: any } }) => {
console.log(e); //this will log { "route": "page1" } when clicked on the first shortcut
};