Error Handling
In this section we'll look at how to handle errors in our application.
Basicly, in app.ts
we'll use the Application.on('unhandledError', (e: UnhandledError) => {}
event to handle errors in iOS and Android.
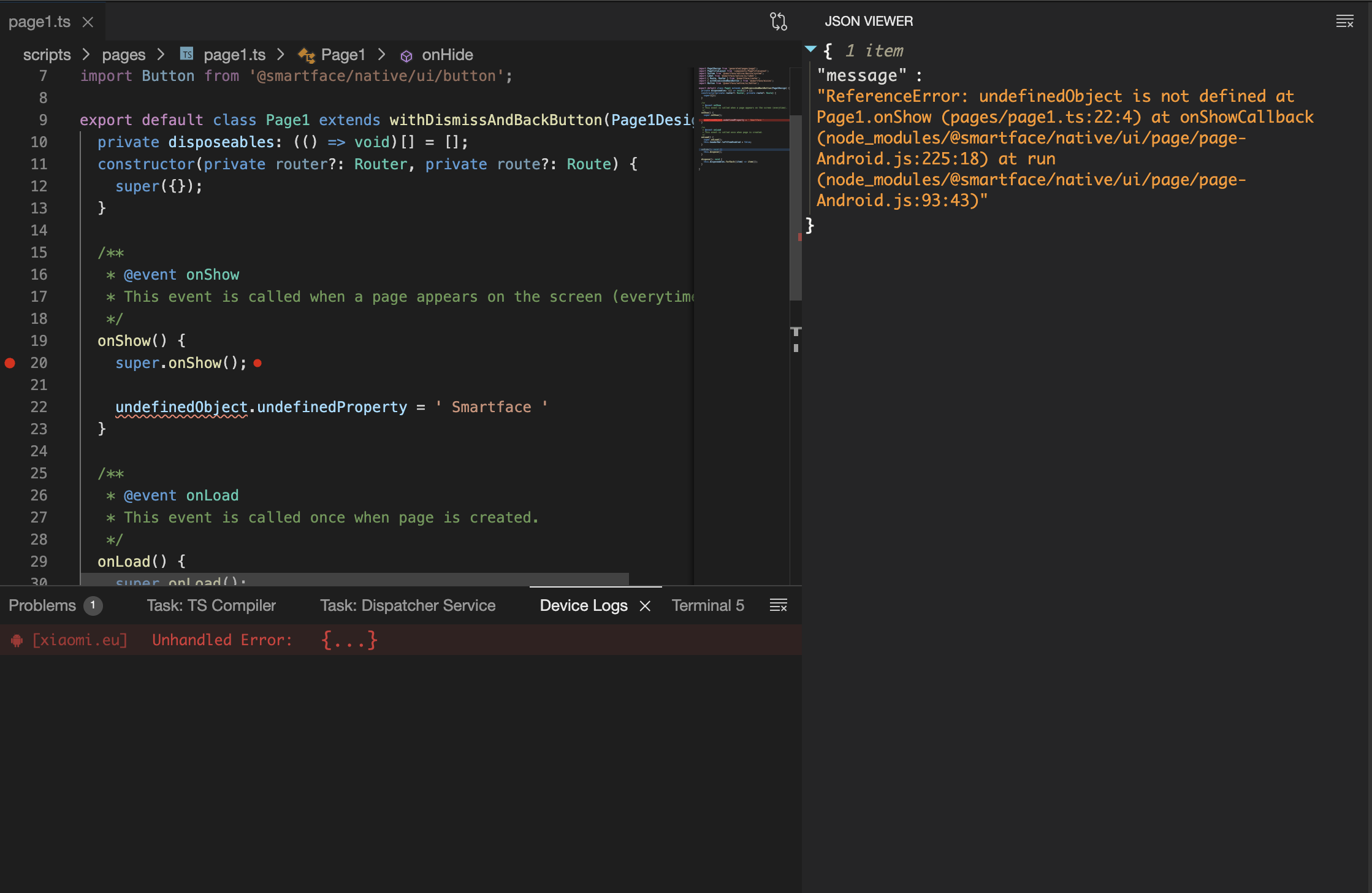
How it works
Application.on('unhandledError', (e: UnhandledError) => {
const error = errorStackBySourceMap(e);
const message = {
message: System.OS === System.OSType.ANDROID ? error.message : e.message,
stack: error.stack
};
if (message.stack) {
console.error('Unhandled Error: ', message);
alert(JSON.stringify(message, null, 2), e.type || 'Application Error');
}
});
Application.on('unhandledError', (e: UnhandledError) => {}
is triggered when an error occurs in iOS or Android.
If that event is not assigned, the error will be handled by Emulator instead.
With assigned :
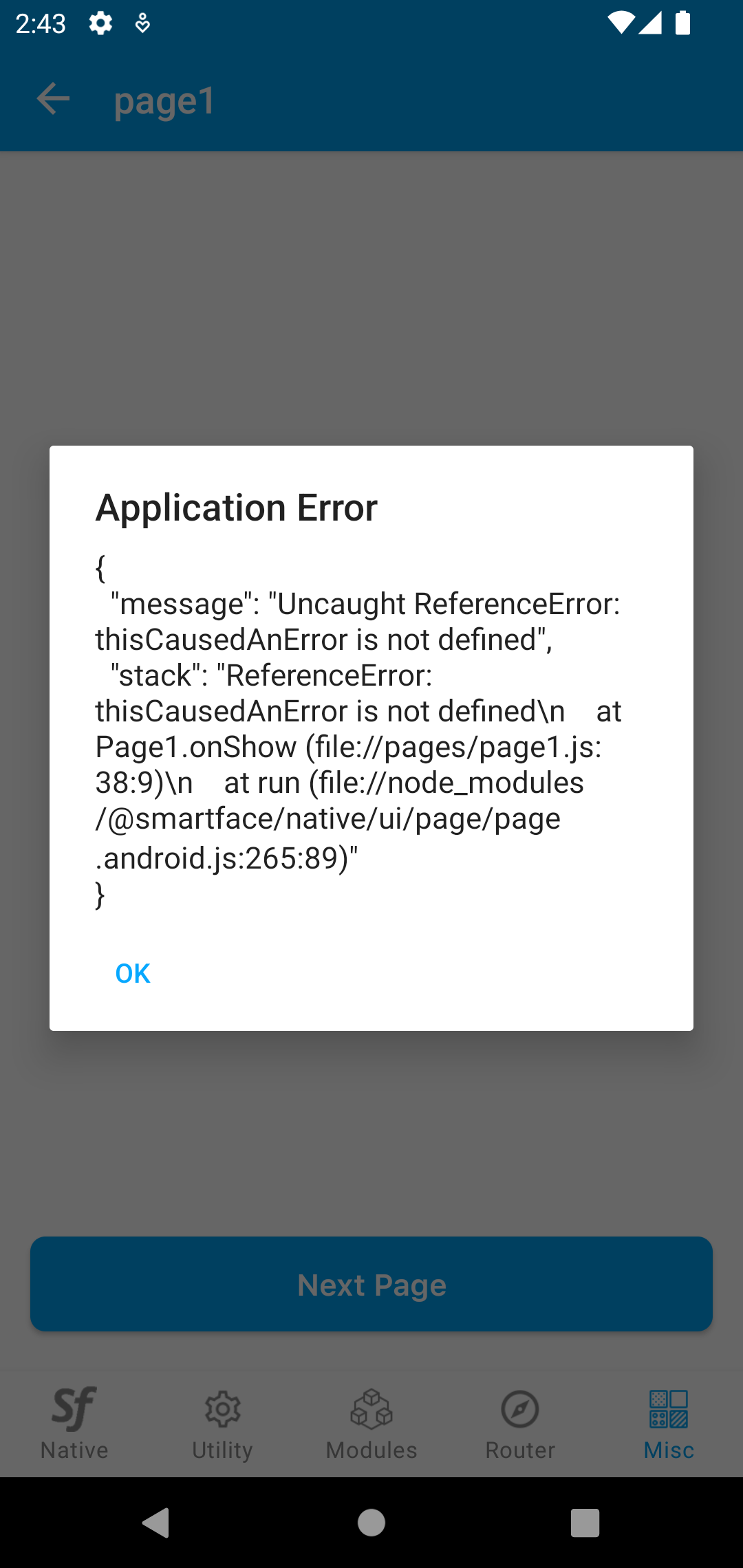
Without assigned :
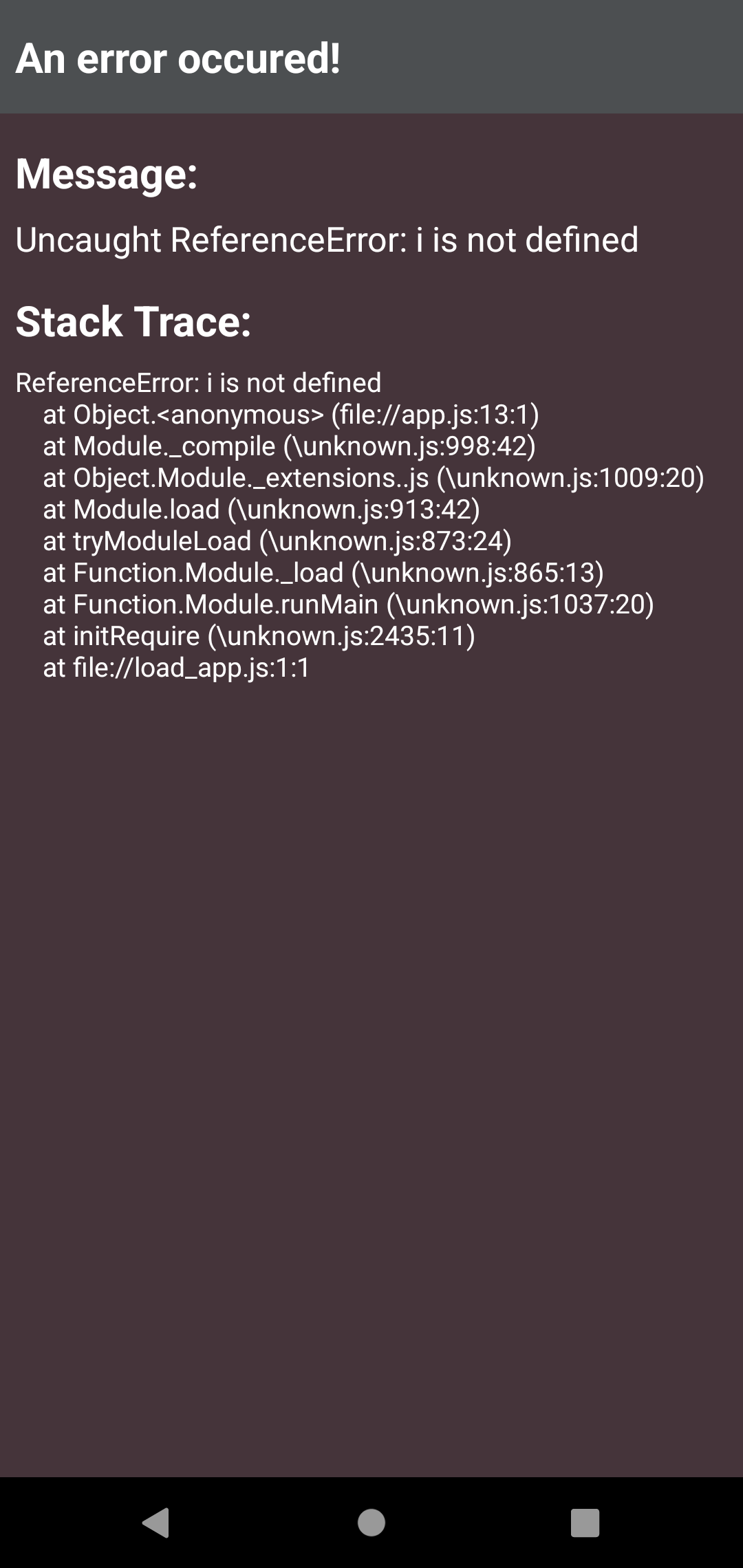
Source Map
In Smartface, Typescript is used as a supported language. Since Typescript is superset of Javascript, the code needs to be compiled to JavaScript in order for mobile application to work. Due to the fact that mobile application reads the Javascript code, error stack will show the Javascript stack by default, which will be useless for us.
In order for error handler to emit the Typescript output, we can use sourceMap
feature included in the Typescript, configurable on tsconfig.json
.
For more information about Source Map, refer to the Typescript documentation:
https://www.typescriptlang.org/tsconfig#sourceMap
On Smartface Projects, sourceMap
value in tsconfig.json
is assigned to true by default.
For more information about Project Architecture, refer to the doc:
Project ArchitectureExample Error Handling Code
On Smartface, sourcemap conversion is done by using the @smartface/source-map module, which is needed to handle the iOS and Android stack logs separately.
Simply send the incoming error to the errorStackBySourceMap
function in order to get the source mapped error stack.
Application.on(Application.Events.UnhandledError, (e: UnhandledError) => {
const error = errorStackBySourceMap(e);
//do stuff with the error
}
This code is taken from helloworld-boilerplate
sample project. The content might subject to change over time.
Application.on(Application.Events.UnhandledError, (e: UnhandledError) => {
const error = errorStackBySourceMap(e);
const message = {
message: System.OS === System.OSType.ANDROID ? error.stack : e.message,
stack: System.OS === System.OSType.IOS ? error.stack : undefined,
};
console.error("Unhandled Error: ", message);
alert(JSON.stringify(message, null, 2), e.type || lang.applicationError);
});
The errors can be seen from the device logs. For more information about device logs, please refer to the device logs documentation:
Device LogsHow to Reopen Device Logs Panel
Device logs panel will be closed at first but automatically open when a device log is emitted by the Dispatcher. If you want to open the Device Logs panel manually or if you accidentally closed it, you can reopen it by Toggle Smartface Device Logs View command.
The default shortcut of Toggle Smartface Device Logs View is ctrl(cmd) + shift +o