EmailComposer
API Reference: UI.EmailComposer
Basic EmailComposer
It is possible to send emails without leaving the app. EmailComposer comes for help for this case.
import PageSampleDesign from 'generated/pages/pageSample';
import FlexLayout from '@smartface/native/ui/flexlayout';
import Application from '@smartface/native/application';
import System from '@smartface/native/device/system';
import File from '@smartface/native/io/file';
import FileStream from '@smartface/native/io/filestream';
import EmailComposer from '@smartface/native/ui/emailcomposer';
import { Route, Router } from '@smartface/router';
import { withDismissAndBackButton } from '@smartface/mixins';
//You should create new Page from UI-Editor and extend with it.
export default class Sample extends withDismissAndBackButton(PageSampleDesign) {
emailcomposer: EmailComposer;
constructor(private router?: Router, private route?: Route) {
super({});
}
// The page design has been made from the code for better
// showcase purposes. As a best practice, remove this and
// use WYSIWYG editor to style your pages.
centerizeTheChildrenLayout() {
this.dispatch({
type: "updateUserStyle",
userStyle: {
flexProps: {
flexDirection: 'ROW',
justifyContent: 'CENTER',
alignItems: 'CENTER'
}
}
})
}
onShow() {
super.onShow();
const { headerBar } = System.OS === System.OSType.ANDROID ? this : this.parentController;
Application.statusBar.visible = false;
headerBar.visible = false;
}
onLoad() {
super.onLoad();
this.centerizeTheChildrenLayout();
//@ts-ignore
if (EmailComposer.canSendMail()) {
this.emailcomposer = new EmailComposer();
this.emailcomposer.setBCC(["bcc@smartface.io", "bcc2@smartface.io"]);
this.emailcomposer.setCC(["cc@smartface.io", "cc2@smartface.io"]);
this.emailcomposer.setTO(["to@smartface.io", "to2@smartface.io"]);
this.emailcomposer.setMessage("message");
this.emailcomposer.setSubject("subject");
this.emailcomposer.onClose = (): void {
console.log("onClose");
};
var imageFile = new File({
path: 'images://smartface.png'
});
this.emailcomposer.android.addAttachmentForAndroid(imageFile);
if (System.OS == "iOS") {
var imageFileStream = imageFile.openStream(FileStream.StreamType.READ, FileStream.ContentMode.BINARY);
var fileBlob = imageFileStream.readToEnd();
imageFileStream.close();
this.emailcomposer.ios.addAttachmentForiOS(fileBlob, "image/png", "smartface.png");
}
this.emailcomposer.show(this);
}
}
}
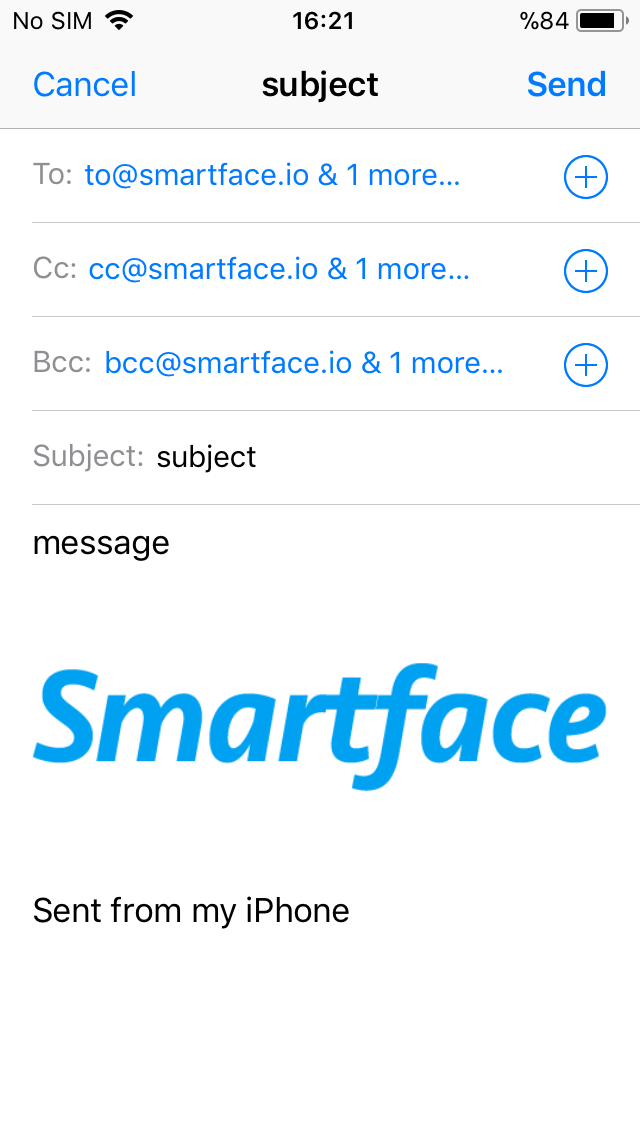
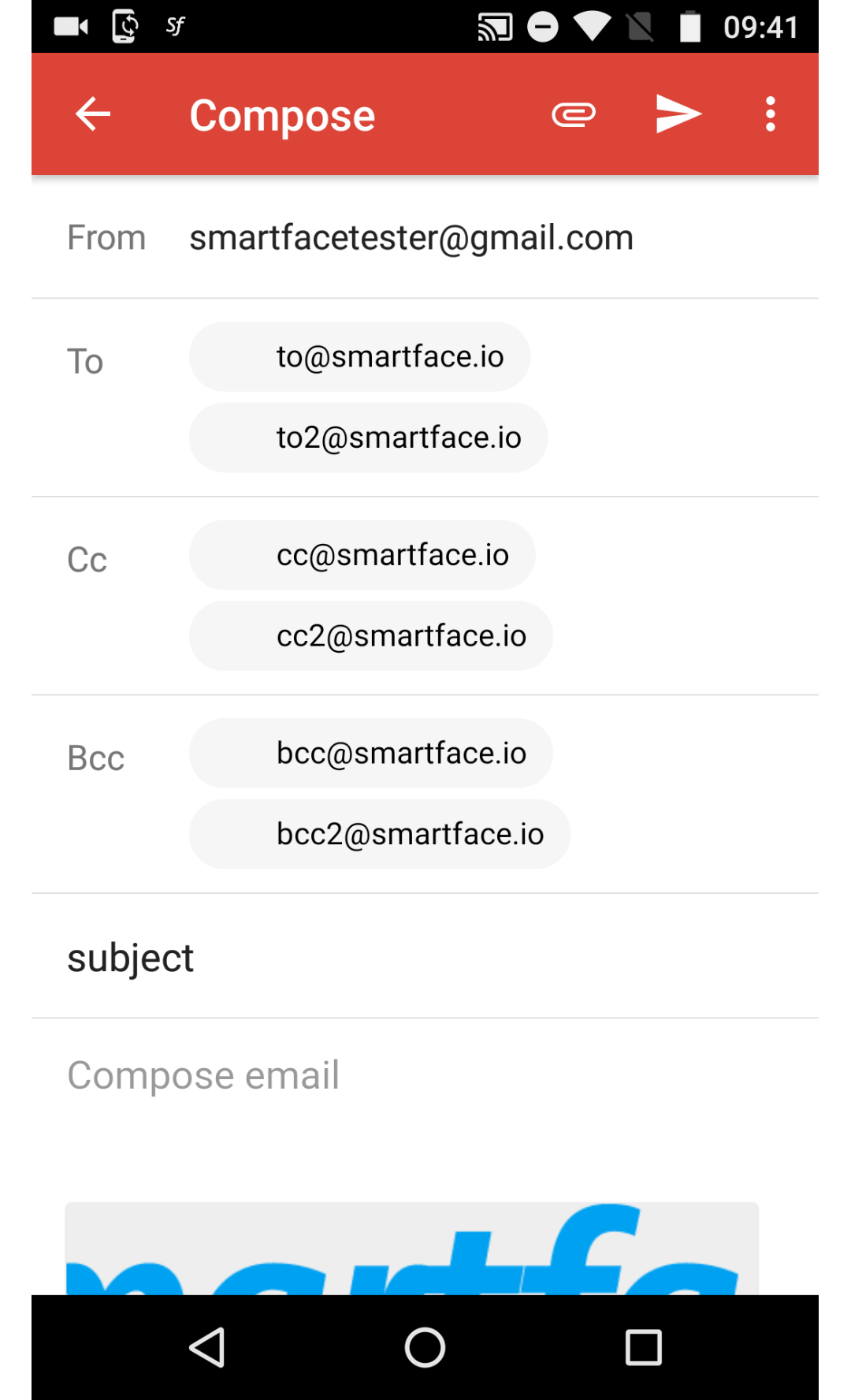
Sending Email with Inter-app Communication
Sending e-mail with leaving the app is also possible with inter-app communication. Check down below for sample code.
Application.call("mailto:info@smartface.io");
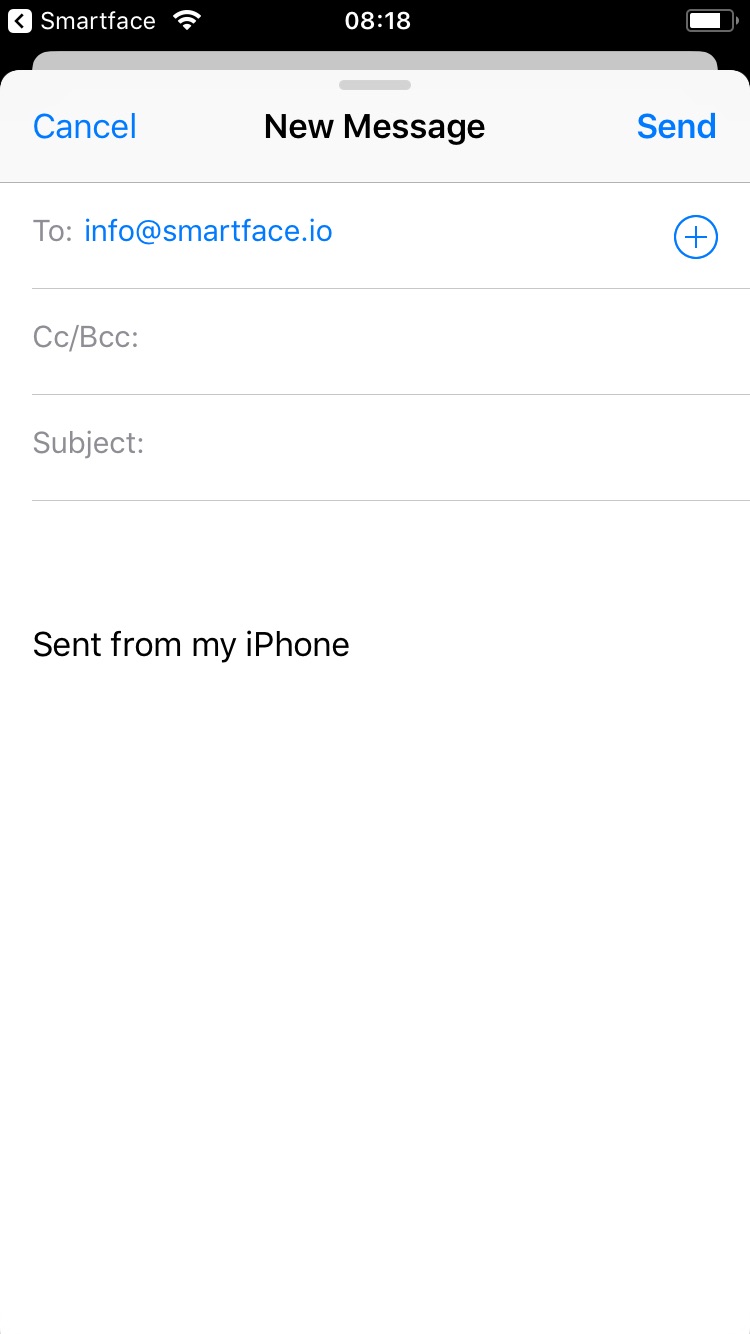
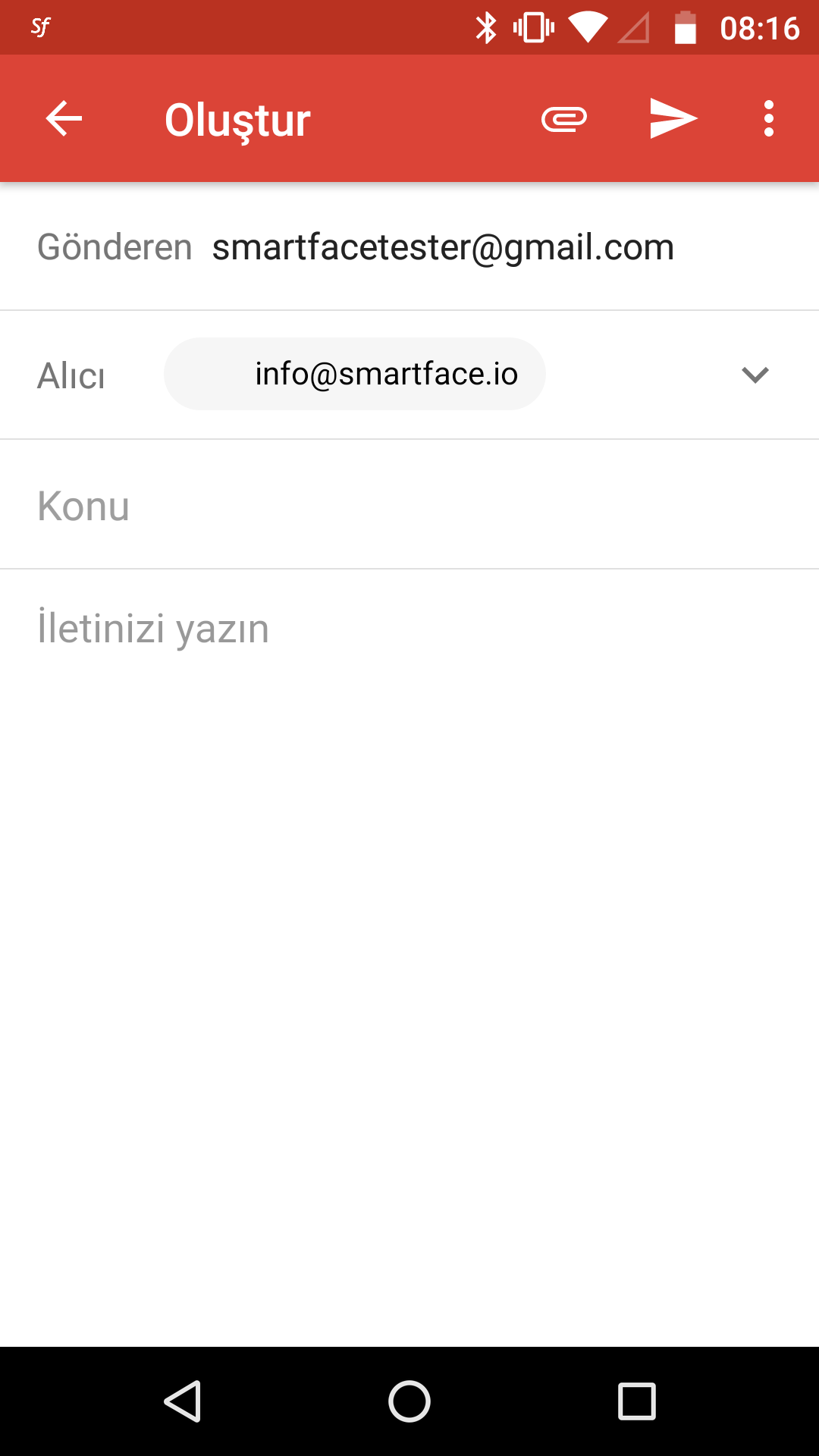