Show Native Prompt
In this section, we'll look at how to use the Alert component to display messages to the user.
Alert is wraps the standard AlertView and simplifies its usage.
Difference between Alert and AlertView
Alert wraps AlertView module and provides more functionality for displaying alerts.
When to use Alert
Alerts are used to display messages to the user and are generally used to inform the user of something that has happened.
Alerts can be used to get user approval or to display errors.
Main use cases for alerts are:
User approval
Errors
Success
Warnings
info
If you need a user prompt to get input from the user inside an alert, please use the AlertView component.
Sort Alert Buttons
- To sort the POSITIVE and NEGATIVE buttons, the last button in the
buttons
array is the first button in theAlert
.
Page
import Page1Design from 'generated/pages/page1';
import PageTitleLayout from 'components/PageTitleLayout';
import { Route, BaseRouter as Router } from '@smartface/router';
import { withDismissAndBackButton } from '@smartface/mixins';
import Button from '@smartface/native/ui/button';
import AlertView from '@smartface/native/ui/alertview';
export default class Page1 extends withDismissAndBackButton(Page1Design) {
private disposeables: (() => void)[] = [];
constructor(private router?: Router, private route?: Route) {
super({});
this.disposeables.push(
// This button is added from UI editor
this.btnNext.on('press', () => {
alert({
title: 'Delete',
message: 'Are you sure you want to delete this item?',
buttons: [
{
text: 'Cancel',
type: AlertView.Android.ButtonType.NEGATIVE,
onClick: () => {
// cancelFunction();
}
},
{
text: 'Delete',
type: AlertView.Android.ButtonType.POSITIVE,
onClick: () => {
// deleteFunction();
}
}
]
});
})
);
}
/**
* @event onShow
* This event is called when a page appears on the screen (everytime).
*/
onShow() {
super.onShow();
}
/**
* @event onLoad
* This event is called once when page is created.
*/
onLoad() {
super.onLoad();
this.headerBar.leftItemEnabled = false;
}
onHide(): void {
this.dispose();
}
dispose(): void {
this.disposeables.forEach((item) => item());
}
}
For more information and usage, please refer to the following documentation:
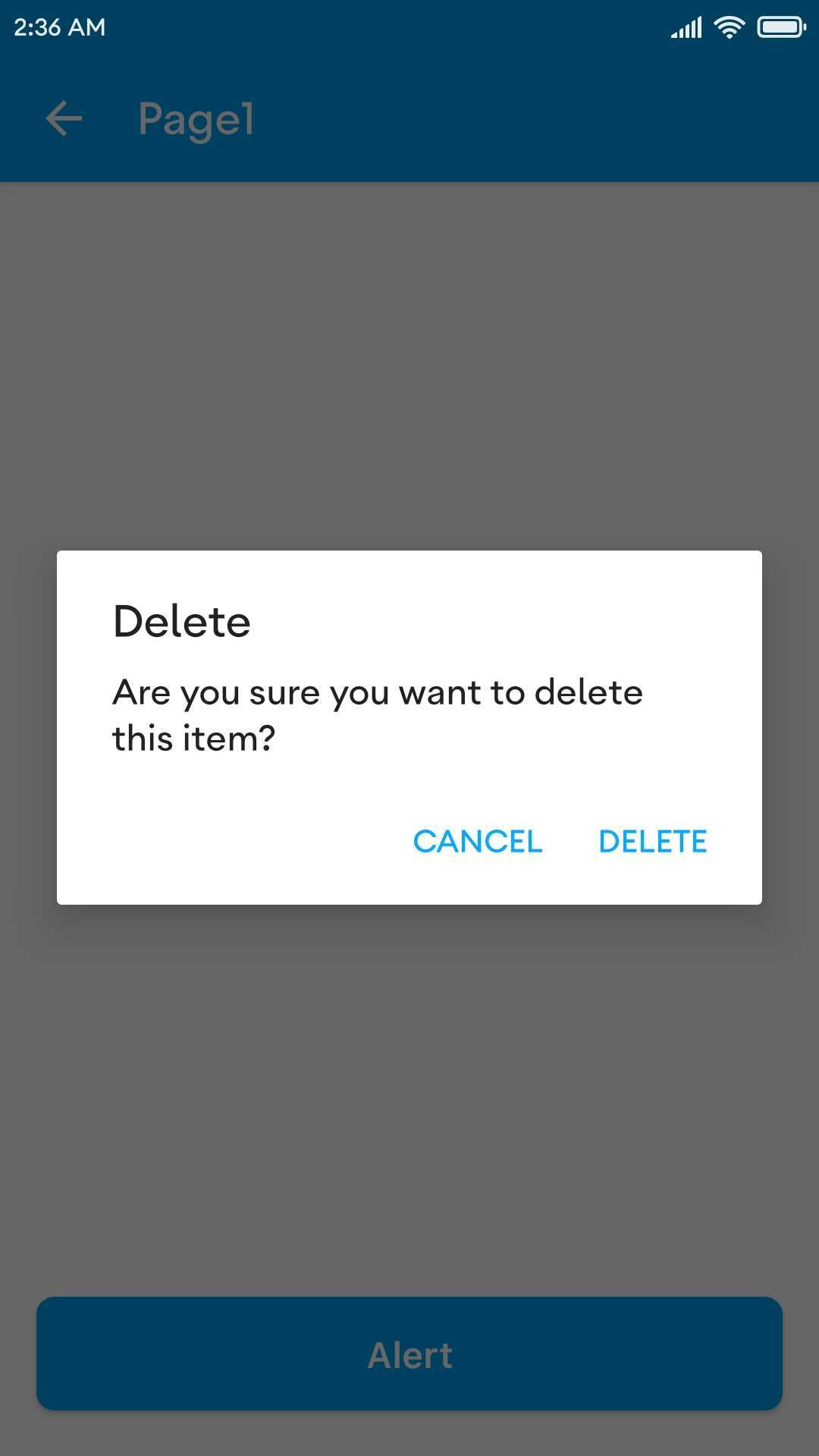
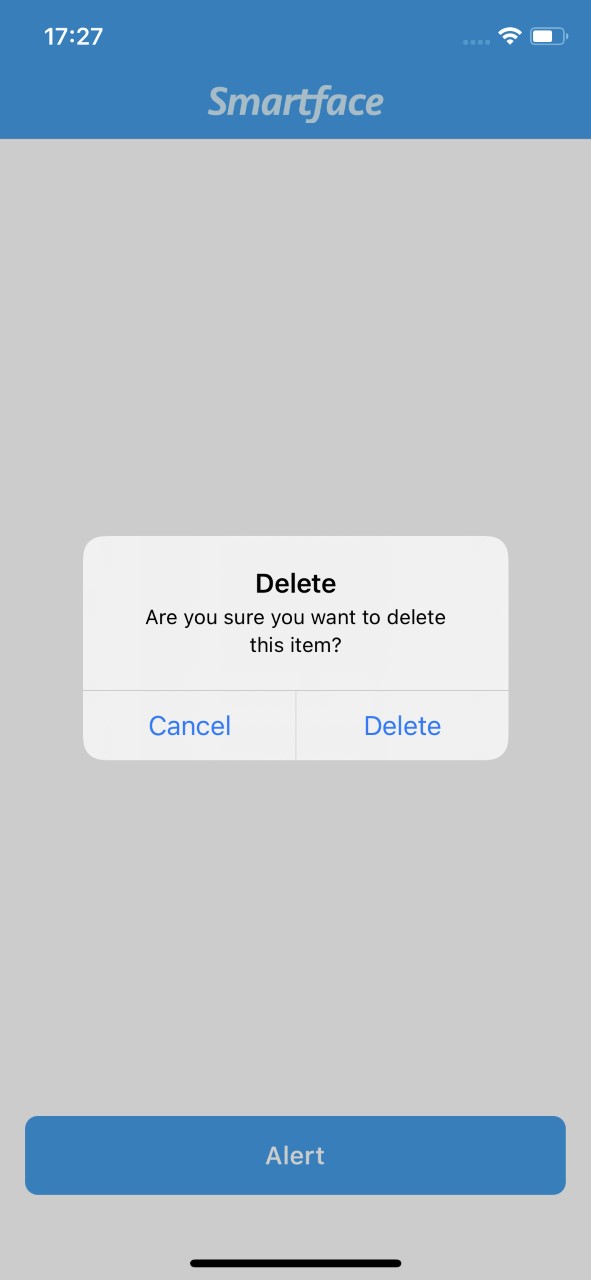