Test Automation
As an application grows, it is eventually going to be cumbersome to manually check every module or page within the application. In that case, test automation tools and methodology can become quite handy.
Since Smartface is a cross-platform mobil platform, it has a unique way to prepare your application to be compatible with any test automation tool.
Assigning Test ID
As you are aware, any underlying component or element prefer a unique testId to ensure that element will be reachable no matter where it is located. Using xpath or navigating through the children is not recommended by any automation tool, since it can be changed.
Points of Interest
The mainline difference is: on iOS, testId is assigned on runtime. On Android, testId is assigned on build time. Therefore, the components and their corresponding ID naming should be defined before the project is built.
- testId should be unique across the whole project. If a testId is not unique, both components will not appear in your test automation tool.
- You can have same component name in different pages, but their testId should not be the same.
- Your testId assignments should not contain whitespaces. Test automation tools might have trouble reading them.
Please keep in mind that testId should not be changed unless it is absolutely necessary. The developers that write testing scripts will rely on the id. Therefore, changing it will break their scripts.
Testing Your Changes
Since Android generates ID fields on build time and iOS assigns them on runtime, your testing process will vary between iOS & Android.
- On iOS, you can test ID assignment directly from the Smartface Emulator.
- On Android, you can not test the ID assignment on the Smartface Emulator. You have to build your application and test it on
.apk
or.aab
file.
If you want to test your changes on iOS simulator, you need to manually extract the .app
file of the application.
Test ID in Smartface UI Editor Components
By default, the components or elements that are created from Smartface UI Editor already have a Test ID that is automatically generated and assigned to them.
This ID will be generated only once and will not change through the project. It can only be changed manually from the Smartface UI editor.
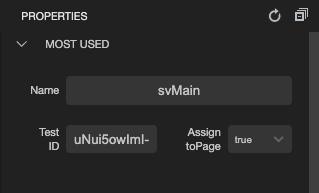
You should take a look at the component structure and add it manually to the ids.xml
file. This file is autogenerated to contain elements created from Smartface UI Editor and it can also be edited manually for components added on runtime.
<?xml version="1.0" encoding="utf-8"?>
<resources>
...
<item name="_Page1_WrapperLayout_ActionButton" type="id" />
<item name="_Page1_WrapperLayout" type="id" />
</resources>
More information can be found below about Smartface UI Editor:
Using Style and ClassesTest ID in Components Created from Code
API Reference: testId
This method is not considered best practice. It is recommended to use Smartface UI Editor unless it is a project-wide component.
When a component is not created from UI editor, it will not have testId assigned automatically. You should manually set it.
You can set testId anytime you want within the Page or Component Life-Cycle. However, to keep the code clean, it is recommended to set testId at onLoad event or immediately after the element is created.
This runtime assignment will only work for iOS. On Android, your component testId fields should be defined before it is added to the project on ids.xml
file. ****
To have more information about page life cycle, please refer the document below:
Page Life-CycleIn the example, the manually created component is also assigned to context. To get more information about context, refer the document below:
Using Style and ClassesIn this example, we have two elements called wrapperLayout and actionButton. actionButton is a child of wrapperLayout, therefore it should defined that way in ids.xml file.
import FlexLayout from "@smartface/native/ui/flexlayout";
import Button from "@smartface/native/ui/button";
function createActionButton() {
const wrapperLayout = new FlexLayout();
const actionButton = new Button();
//@ts-ignore
this.layout.addChild(flexLayout1, "wrapperLayout", ".sf-flexLayout");
//@ts-ignore
wrapperLayout.addChild(actionButton, "actionButton", ".sf-button");
this.wrapperLayout = wrapperLayout;
this.actionButton = actionButton;
this.wrapperLayout.testId = "_Page1_WrapperLayout"; // Name should be unique across the project.
this.actionButton.testId = "_Page1_WrapperLayout_ActionButton"; // Name should be unique across the project.
}
The names should be exactly same with how it is defined at ids.xml
file to not be different
Unlike while adding the layout to the page with addChild method, you should also note which parent it belongs.
Test ID in ListView or GridView
Since listView or GridView uses RecyclerView method, the developer needs to implement testId in a way that it will not overlap and keep the uniqueness across the project.
ListView or GridView repeats the same component to gain performance and elements inside of them cannot be distinguished without any tweaks.
To learn about ListView or GridView in general, refer to the document below:
ListViewSince the items inside will repeat, you need to access the elements inside within the test automation tool. Please refer to the document below to access your ListView or GridView content:
Integrating Test Automation on SmartfaceHere is an iOS only example using ListView and assigning relative testId:
// Assuming listView1 was created from Smartface UI Editor
function initListView() {
this.listView1.onRowBind = (listviewItem, index) => {
// Your data binding goes here
listViewItem.testId = `page1/listView1/item-${index}`;
};
}
This method will only work on iOS. Only use this for a specific course of action.
To ensure uniqueness, index was used to distinguish between components. Another field like title or any other property can be used, as long as it is guaranteed that they are unique.
More information about onRowBind usage can be found below:
Data Binding