VideoView
API Reference: UI.VideoView
VideoView is a view to play video clips. Supported formats for both platforms are 3GPP (.3gp) and MPEG-4 (.mp4).
The components in the example are added from the code for better showcase purposes. To learn more about the subject you can refer to:
Adding Component From CodeAs a best practice, Smartface recommends using the WYSIWYG editor in order to add components and styles to your page or library. To learn how to use UI Editor better, please refer to this documentation
UI Editor Basicsimport PageSampleDesign from "generated/pages/pageSample";
import { Route, Router } from "@smartface/router";
import { styleableComponentMixin } from "@smartface/styling-context";
import Application from "@smartface/native/application";
import VideoView from "@smartface/native/ui/videoview";
class StyleableVideoView extends styleableComponentMixin(VideoView) {}
//You should create new Page from UI-Editor and extend with it.
export default class Sample extends PageSampleDesign {
myVideoView: StyleableVideoView;
constructor(private router?: Router, private route?: Route) {
super({});
}
// The page design has been made from the code for better
// showcase purposes. As a best practice, remove this and
// use WYSIWYG editor to style your pages.
centerizeTheChildrenLayout() {
this.dispatch({
type: "updateUserStyle",
userStyle: {
flexProps: {
flexDirection: "ROW",
justifyContent: "CENTER",
alignItems: "CENTER",
},
},
});
}
onShow() {
super.onShow();
const { headerBar } = this;
Application.statusBar.visible = false;
headerBar.visible = false;
}
onLoad() {
super.onLoad();
this.centerizeTheChildrenLayout();
this.myVideoView = new StyleableVideoView({
onReady: () => {
this.myVideoView.play();
},
});
this.myVideoView.ios.page = this;
this.myVideoView.loadURL("http-video-url");
this.addChild(this.myVideoView, "myVideoView", ".sf-videoView", {
margin: 20,
height: 250,
width: 250,
});
}
}
iOS provides controller bar for videos unlike Android.
You need to add some objects(like button or label) to get the same feature only for Android.
You should not forget to call the 'pause' or 'stop' method before the page is hidden.
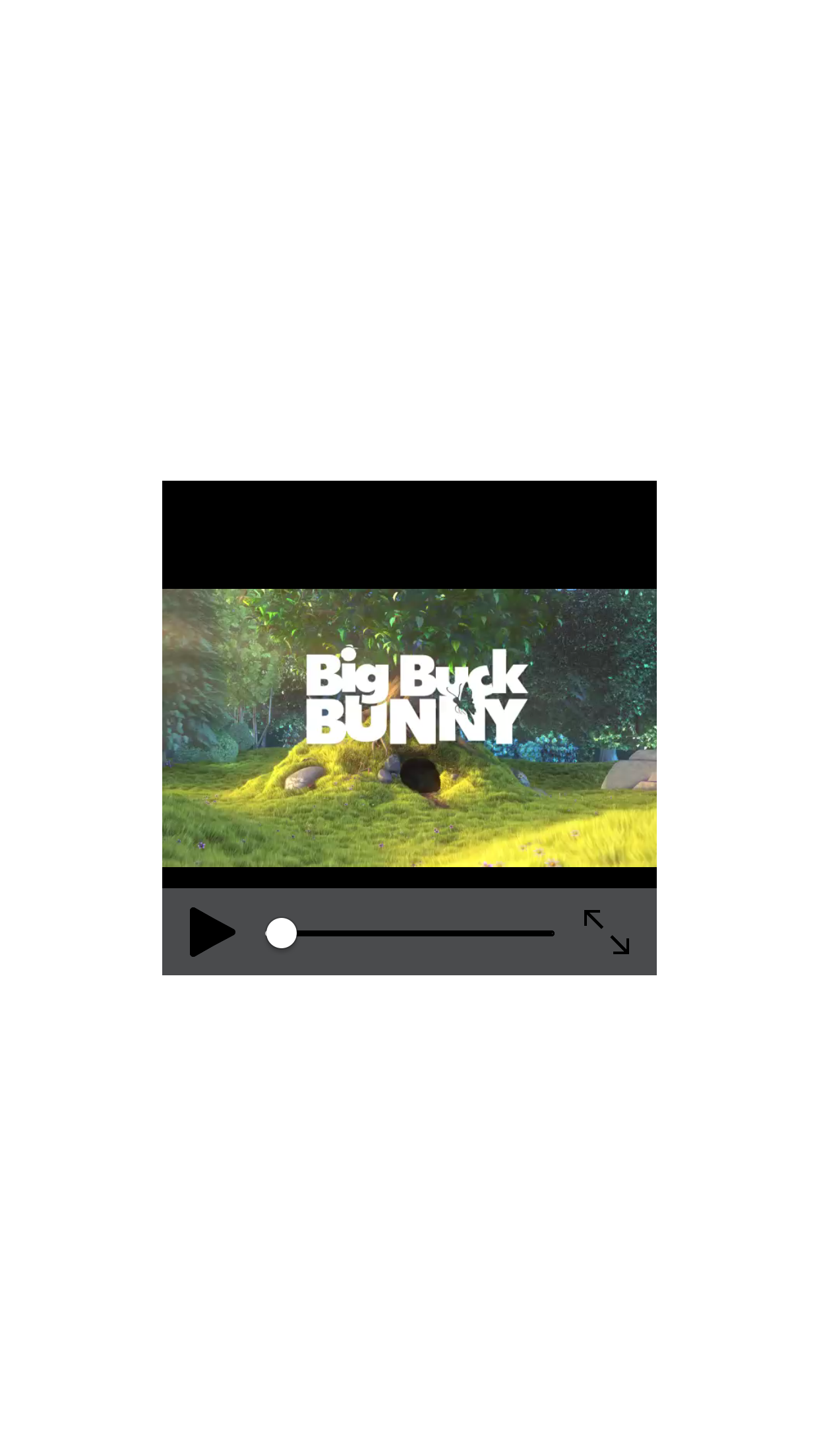
Fullscreen on Android
Android do not directly support fullscreen mode for VideoView. Adjusting the layout of the page is the only way to achieve fullscreen mode.
import Page1Design from "generated/pages/page1";
import Router from "@smartface/router/lib/router/Router";
import { Route } from "@smartface/router";
import { withDismissAndBackButton } from "@smartface/mixins";
import Page from "@smartface/native/ui/page";
import System from "@smartface/native/device/system";
import Screen from "@smartface/native/device/screen";
import VideoView from "@smartface/native/ui/videoview";
import FlexLayout from "@smartface/native/ui/flexlayout";
import { styleableComponentMixin } from "@smartface/styling-context";
class StyleableFlexLayout extends styleableComponentMixin(FlexLayout) {}
class StyleableVideoView extends styleableComponentMixin(VideoView) {}
export default class Page1 extends withDismissAndBackButton(Page1Design) {
video: StyleableVideoView;
flImage: StyleableFlexLayout;
constructor(private router?: Router, private route?: Route) {
super({});
this.onOrientationChange = ({ orientation }) => {
if (System.OS === System.OSType.ANDROID) {
let newHeight = Screen.height;
if (orientation !== Page.Orientation.PORTRAIT) {
this.headerBar.visible = false;
this.statusBar.visible = false;
} else {
newHeight = this.flImage.height;
this.headerBar.visible = true;
this.statusBar.visible = true;
this.orientation = Page.Orientation.AUTO;
}
this.flImage.dispatch({
type: "updateUserStyle",
userStyle: {
height: newHeight,
},
});
}
};
}
initVideoView() {
this.video = new StyleableVideoView();
this.video.loadURL(
"https://commondatastorage.googleapis.com/gtv-videos-bucket/sample/BigBuckBunny.mp4"
);
this.video.android.onFullScreenModeChanged = (isFullScreen) => {
if (isFullScreen) {
this.orientation = Page.Orientation.AUTOLANDSCAPE;
setTimeout(() => (this.orientation = Page.Orientation.AUTO), 2500);
} else {
this.orientation = Page.Orientation.PORTRAIT;
}
};
this.flImage.addChild(this.video, "video", ".sf-videoView");
}
initFlexLayout() {
this.flImage = new StyleableFlexLayout();
this.addChild(this.flImage, "flImage", ".sf-flexLayout");
}
onShow() {
super.onShow();
this.initBackButton(this.router);
}
onLoad() {
super.onLoad();
this.initFlexLayout();
this.initVideoView();
}
}