SMS Handling
On Mobile Devices, there will be the time where you will need to handle incoming SMS message for various of reasons exampled below:
- Auto-filling the SMS verification code
- Streaming the SMS messages to your app ( Android only )
- Creating a built-in SMS reader ( Android only )
Apple doesn't allow any 3rd party software to read the SMS messages from any Apple device.
Handle incoming SMS on Android
To read the SMS contents of your phone, refer to SMS Receiver. Since Android doesn't support out of the box 2FA handling, developers should implement their own views to handle SMS autofill.
import SMSReceiver from '@smartface/native/device/smsreceiver';
import { PermissionResult, Permissions } from '@smartface/native/device/permission/permission';
Permission.android.requestPermissions(Permissions.ANDROID.RECEIVE_SMS).then((e) => {
const result = e[0];
if (result === PermissionResult.GRANTED) {
SMSReceiver.registerReceiver();
SMSReceiver.callback = (e) => {
console.info(e);
alert('SMS IS RECEIVED');
SMSReceiver.unRegisterReceiver();
};
}
});
Handle incoming SMS on iOS
As hinted above, there is no supported way to read the contents directly on an iOS. However, starting with iOS 12, it is possible to get verification code sent to iMessage on your application.
It is not possible to get SMS content of an iPhone using iOS 11 or below.
By default, Smartface TextBoxes or MaterialTextBoxes may receive OTP without any configuration. However, it is strongly advised to include textContentType to your code.
Refer to TextContentType API
import TextContentType from "@smartface/native/ui/textcontenttype";
import System from "@smartface/native/device/system";
function initTextBox() {
if (System.OS === System.OSType.ANDROID) {
return;
}
//TextBox
this.textBox1.ios.textContentType = TextContentType.ONETIMECODE;
//MaterialTextBox
this.mtbLogin.materialTextBox.ios.textContentType =
TextContentType.ONETIMECODE;
}
The OTP will only be shown if the textbox field is empty.
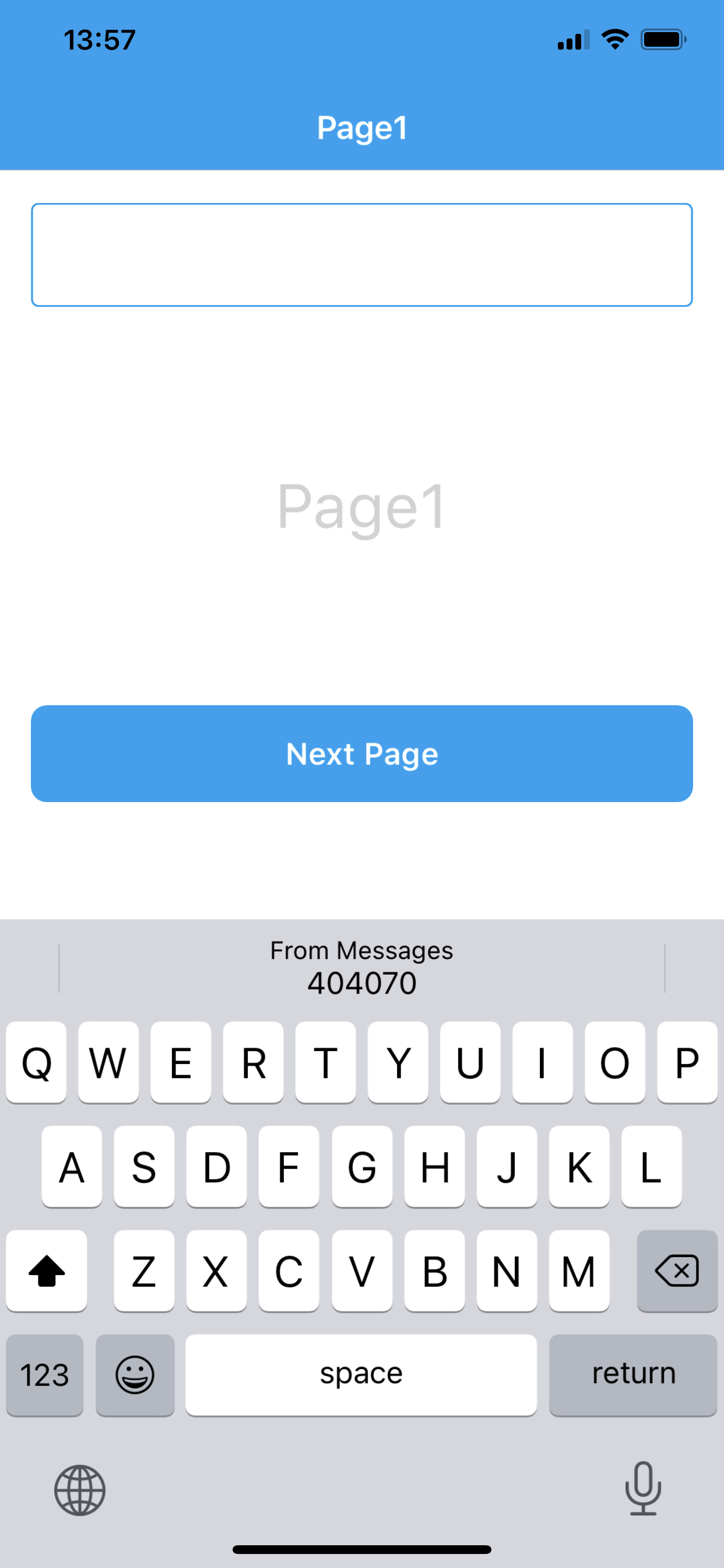
If you are the SMS provider for the app, refer to this article by Apple to get a gist of how to format your SMS messages for Apple devices to detect.
Some native features might require specific permissions and published app to work on Smartface due to Smartface Android Emulator itself not having necessary permissions.
List of permissions disabled by the emulator:
android.permission.READ_SMS
android.permission.SEND_SMS
android.permission.RECEIVE_SMS
android.permission.RECEIVE_MMS
You can add this permission to your AndroidManifest.xml file to enable the feature on your published app.