Developing Android Plugins
What Is an Android Plugin?
Android plugins are native Android projects that you can bundle with the Smartface Native Framework framework in the publishing step. This enables you use native Android libraries inside Smartface Native Framework.
When you add an Android plugin to a Smartface project, Smartface CLI tool gets resources and classes of the plugin and bundles them with Smartface Native Framework -. Resulting apk file includes all native libraries and plugin libraries together.
For further reading about how you access Native API's inside a Smartface project, you can check this guide.
How It Works?
Smartface Native Framework framework has an engine proxying Javascript calls to the Android runtime. You can read Smartface Native Framework Architecture for more information about the Smartface Native Framework architecture.
This engine loads plugin classes that you bundled with the Smartface CLI, when you require them from the JavaScript side. Then all you need to do is to use your libraries in JavaScript as you would use them in a native Android project.
Below you can find a sample code that uses java.lang.String class from JavaScript.
const StringClass = requireClass("java.lang.String");
let stringInstance = new StringClass("This will create a Java String object");
console.log(stringInstance.toLowerCase());
Plugin Development Prerequisites
Smartface Native Framework Android plugin development requires native Android development environment.
- Download and install Android Studio
- Install Java 1.8 or higher
Plugin Development
In this section, you can find information about how to configure your plugin development environment and start developing your plugin.
We support AAR file, library module and remote binary dependencies.
Create android library module
Please visit android developer guide how to create android library.
Click File -> New -> New Module, then select Android Library.
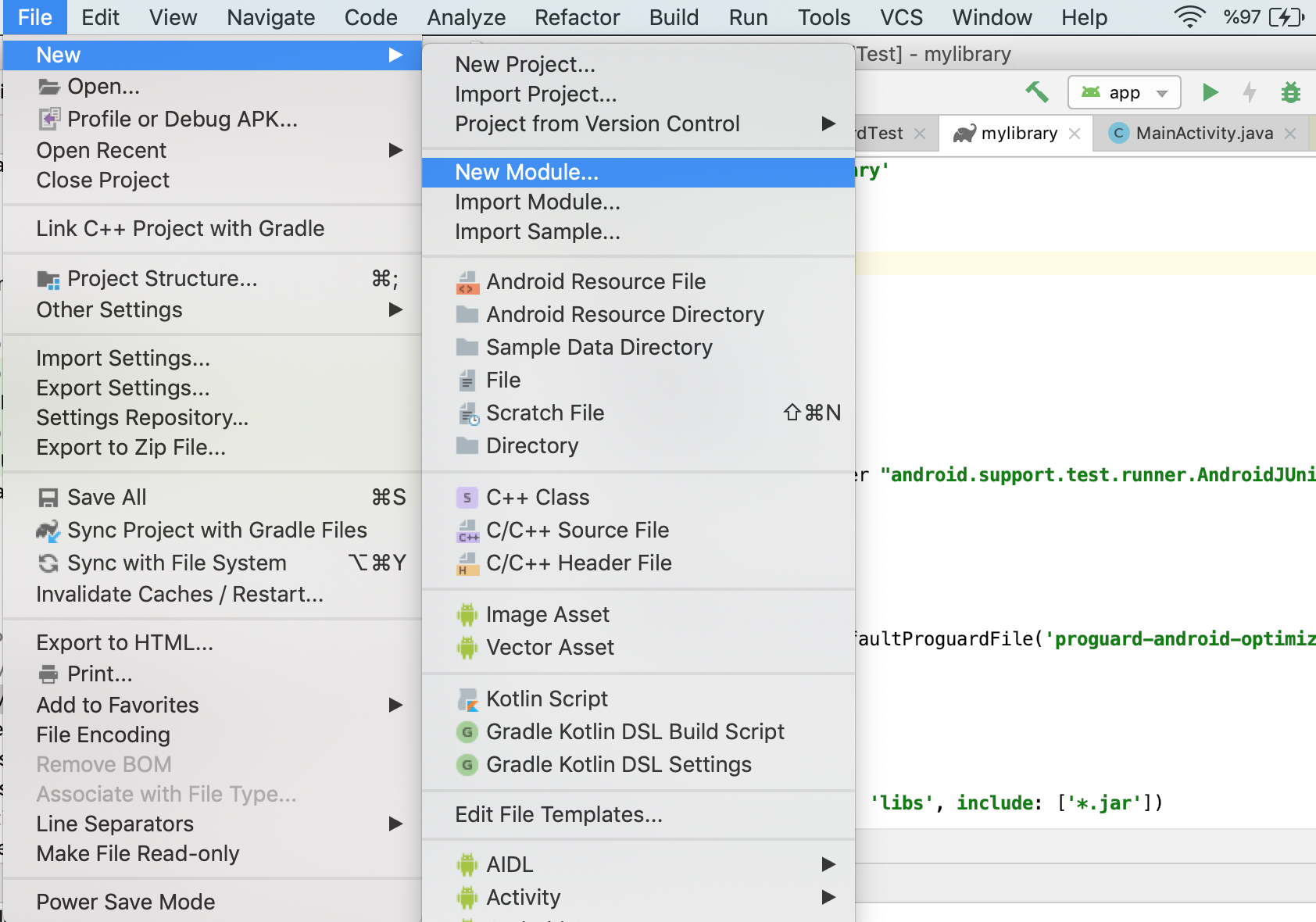
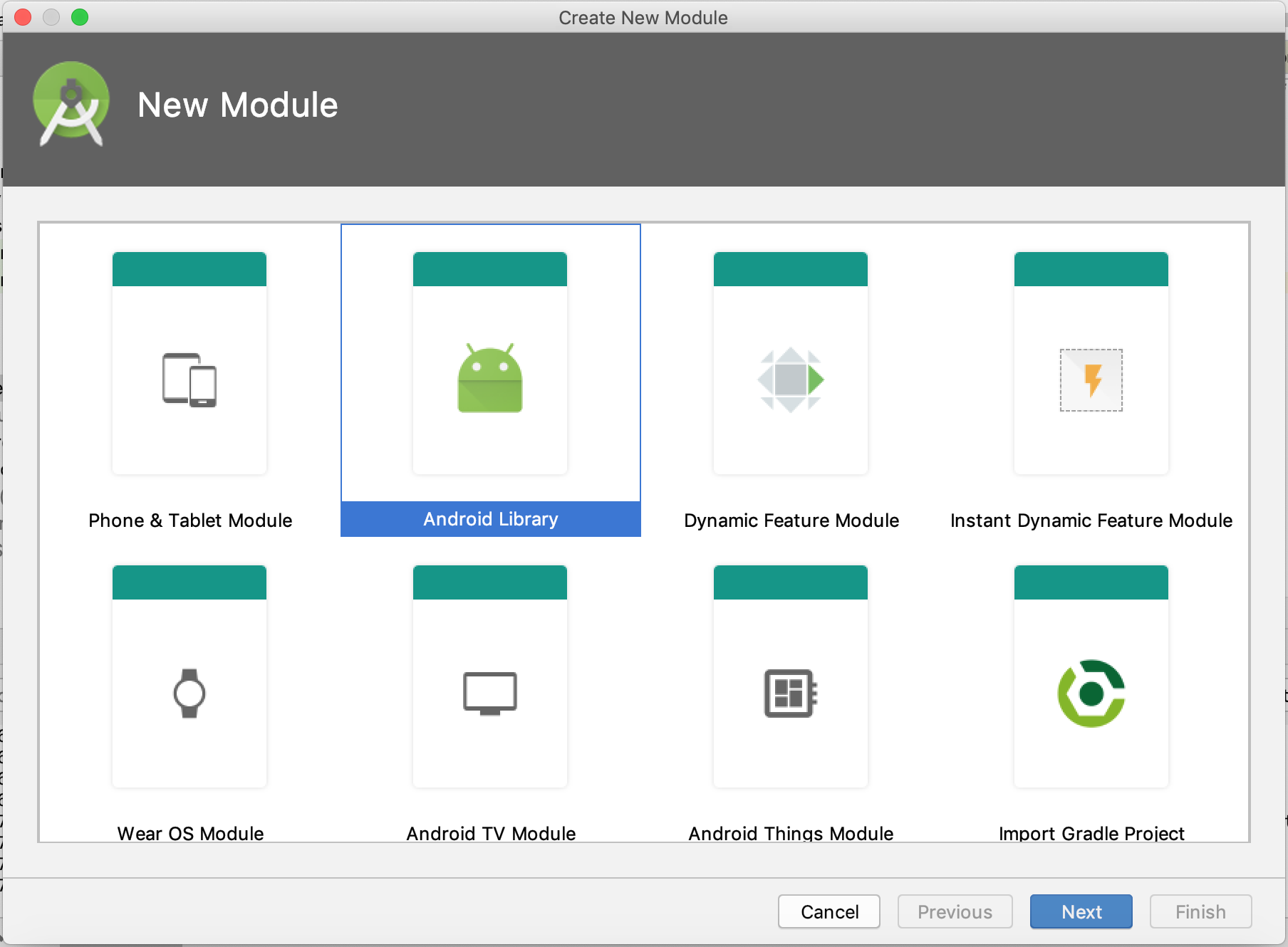
Export android library module as AAR file
When you want to build the AAR file, select the library module in the Project window and then click Build -> Build APK.
An .aar file can include the following types of files:
- /AndroidManifest.xml (mandatory)
- /classes.jar (mandatory)
- /res/ (mandatory)
- /R.txt (mandatory)
- /assets/ (optional)
- /libs/*.jar (optional)
- /jni//*.so (optional)
- /proguard.txt (optional)
- /lint.jar (optional)
Updating build.gradle
Smartface Native Framework has some specific configurations for the build and since your plugin will merge with framework, the plugin must have same configurations as well.
Please check configuration below and be sure you have same configurations in your build.gradle file.
Make sure to set Minimum SDK to API 19 since Smartface Native Framework currently supports minimum API 19.
Smartface Native Framework has been migrated over to AndroidX. You can use AndroidX dependencies.
android {
defaultConfig {
...
minSdkVersion 19
targetSdkVersion 28
multiDexEnabled true
}
...
}
dependencies {
implementation 'com.android.support:multidex:1.0.0'
implementation 'com.android.support:appcompat-v7:28.0.0'
// AndroidX is supported by Smartface Native Framework
// implementation 'androidx.appcompat:appcompat:1.0.0'
}
Smartface supports gradle 3.2.0 build version. In order to build the plugin successfully, we recommend you to use 'com.android.tools.build:gradle:3.2.0' build version.
Building the Project
After setting up your development environment, build your project and run on the device to see if everything is working fine.
You can use AAR file or library module directly on your workspace. Put your plugin on your workspace. To specify dependency's path, edit your project.json file under config.
{
...
"build": {
"android": {
"plugins": {
"aars": {
"aar-name": {
"path": "your-aar-path",
"active": true
}
},
"modules": {
"library-name": {
"path": "your-library-path",
"active": true
}
}
}
}
}
...
}
Edit your dependencies.gradle file under config/Android.
dependencies {
// Dependency on local binaries
implementation(name: 'file-name', ext: 'aar')
// Dependency on a local library module
implementation project(':library-name')
// Dependency on a remote binary
implementation 'com.example.android:app-magic:12.3'
}
You just generated a ready-to-use Smartface Native Framework Android Plugin!
Please follow this link to use your first plugin in a smartface project.
Sample Usage
Let's say that you created the com.packagename.Calculator
class in your Android plugin project as given below.
package com.packagename;
class Calculator {
private float value;
public Calculator(float value) {
this.value = value;
}
public void add(float value) {
this.value += value;
}
public float getValue() {
return this.value;
}
public static float add(float a, float b) {
return a+b;
}
}
If you want to access this Java class from JavaScript, below is a sample code:
const Calculator = requireClass("com.packagename.Calculator");
// Accessing static function
var a = 5,
b = 3;
alert("3+5=" + Calculator.add(3, 5));
// Creating instance and accessing member function
var calculatorIns = new Calculator(7);
calculatorIns.add(12);
alert("7+12=" + calculatorIns.getValue());
While accessing native classes on Android sometimes you will need Context. You can use Android.getActivity() method to access current application context.
Limitations
App Theme
Smartface Native Framework uses ActionBarActivity so it’s a must for the plugin developer to use Theme.AppCompat theme (or descendant) in the plugin project.
Emulator
Smartface Native Framework Android plugins can be used in published projects only. Plugins in Smartface projects will not work in the Smartface on-device emulator.