TabBarController
API Reference: UI.TabBarController
TabBarController organizes and allows navigation between groups of Pages that are related and navigation controller. Pages can be created and added on onPageCreate() function. TabBar indicator shows which bar is selected. Divider helps to seperate TabBars on only Android.
To have a tabbarController page, first you need to create a base page that extends from the TabBarController and the pages for all tabs needs to be returned from the onPageCreate method on the base page.
import PageSampleDesign from "generated/pages/pageSample";
import { Route, Router } from "@smartface/router";
import Application from "@smartface/native/application";
import Color from "@smartface/native/ui/color";
import Image from "@smartface/native/ui/image";
import TabBarItem from "@smartface/native/ui/tabbaritem";
import TabBarController from '@smartface/native/ui/tabbarcontroller';
import { themeService } from "theme";
import Page from "@smartface/native/ui/page";
import PgMessagesDesign from "generated/pages/pgMessages";
import YourPage from 'pages/yourPage';
export default class BaseTabbar extends TabBarController {
tabPages: Page[] = [
new YourPage(),
new YourPage(),
new YourPage(),
new YourPage(),
];
recentsImage: Image = Image.createFromFile("images://recentcall.png");
favImage: Image = Image.createFromFile("images://fav.png");
contactImage: Image = Image.createFromFile("images://contacts.png");
messageImage: Image = Image.createFromFile("images://message.png");
items: TabBarItem[];
constructor(private router?: Router, private route?: Route) {
super({});
let recentItem: TabBarItem = new TabBarItem({
title: "Recent",
icon: this.recentsImage,
});
let favItem: TabBarItem = new TabBarItem({
title: "Favorite",
icon: this.favImage,
});
let contactItem: TabBarItem = new TabBarItem({
title: "Contact",
icon: this.contactImage,
});
let messageItem: TabBarItem = new TabBarItem({
title: "Message",
icon: this.messageImage,
});
this.items = [recentItem, favItem, contactItem, messageItem];
}
onPageCreate(index: number): Page {
return this.tabPages[index];
}
onSelected(index) {
console.log("Selected item index: " + index);
}
onShow() {
super.onShow();
}
onLoad() {
this.headerBar.title = this.constructor.name; //Tabbarcontroller requires manual setup
this.scrollEnabled = true;
this.indicatorColor = Color.BLACK;
this.indicatorHeight = 3;
this.barColor = Color.create("#F3F0F0");
this.textColor = {
normal: Color.BLACK,
selected: Color.create("#00A1F1"),
};
this.autoCapitalize = true;
}
}
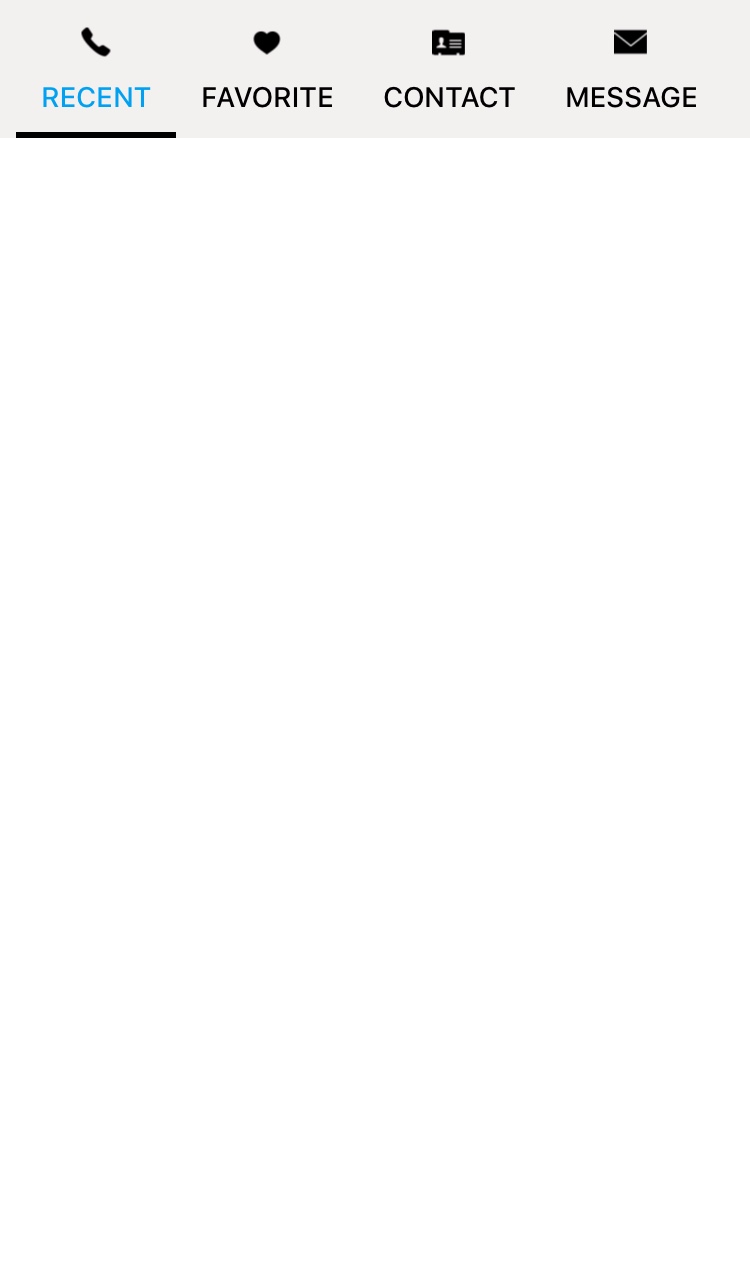
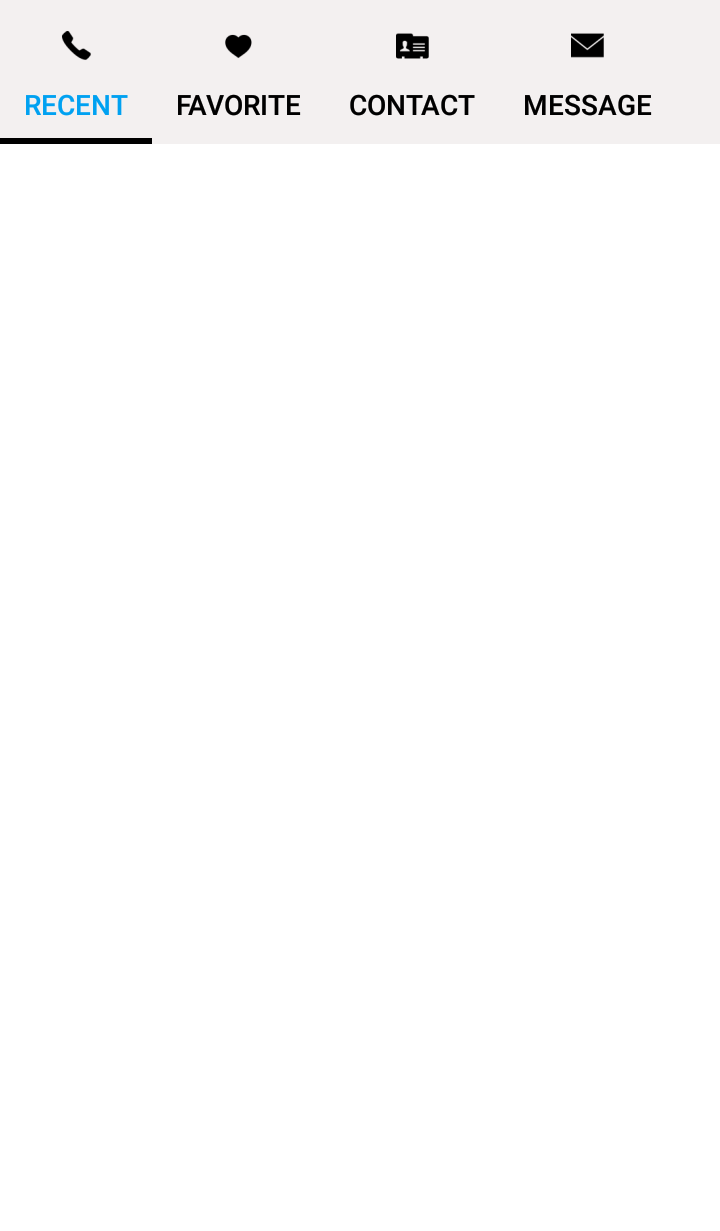
To improve performance, the onPageCreate event is fired differently on Android and iOS. When the page is changed, iOS fires the method for the newly created page but Android fires the onPageCreate event for the second next page as well, calling the method for two pages at the same time.
Since our TabBarController base page is a special page that extended from the TabBarController, some of the contxjs stuff like pushClassNames, removeClassNames will not work.
Known issue; In Android, if lower-case text in the tab items required, the autoCapitalize property must be assigned each time when the tab item's appearance updated.