FlexShrink
API Reference: UI.View.flexShrink
This property specifies how much a view will shrink relative to the other views inside the same FlexLayout. It aims to shrink the view to fit to the screen.
Tip
You need to set wrap property as flexWrap.NOWRAP to observe effects of flexShrink.
note
The components in the example are added from the code for better showcase purposes. To learn more about the subject you can refer to:
Adding Component From CodeAs a best practice, Smartface recommends using the WYSIWYG editor in order to add components and styles to your page or library. To learn how to use UI Editor better, please refer to this documentation
UI Editor Basicsimport PageSampleDesign from "generated/pages/page3";
import { Route, Router } from "@smartface/router";
import Application from "@smartface/native/application";
import { styleableComponentMixin, styleableContainerComponentMixin } from "@smartface/styling-context";
import FlexLayout from "@smartface/native/ui/flexlayout";
import Label from "@smartface/native/ui/label";
class StyleableLabel extends styleableComponentMixin(Label) {}
class StyleableFlexLayout extends styleableContainerComponentMixin(FlexLayout) {}
//You should create new Page from UI-Editor and extend with it.
export default class Sample extends PageSampleDesign {
myFlexLayout: StyleableFlexLayout;
label1: StyleableLabel;
label2: StyleableLabel;
label3: StyleableLabel;
constructor(private router?: Router, private route?: Route) {
super({});
}
// The page design has been made from the code for better
// showcase purposes. As a best practice, remove this and
// use WYSIWYG editor to style your pages.
centerizeTheChildrenLayout() {
this.dispatch({
type: "updateUserStyle",
userStyle: {
flexProps: {
flexDirection: 'ROW',
justifyContent: 'CENTER',
alignItems: 'CENTER'
}
}
})
}
onShow() {
super.onShow();
const { headerBar } = this;
Application.statusBar.visible = false;
headerBar.visible = false;
}
onLoad() {
super.onLoad();
this.centerizeTheChildrenLayout();
this.myFlexLayout = new StyleableFlexLayout();
this.addChild(this.myFlexLayout, "myFlexLayout", ".sf-flexLayout", {
top: 0,
left: 0,
bottom: 0,
right: 0,
flexProps: {
positionType: "ABSOLUTE",
flexDirection: "ROW",
},
backgroundColor: "#FFFFFF",
});
this.label1 = new StyleableLabel({
text: "flexShrink:1",
});
this.label2 = new StyleableLabel({
text: "flexShrink:5",
});
this.label3 = new StyleableLabel({
text: "flexShrink:10",
});
this.myFlexLayout.addChild(this.label1, "label1", ".sf-flexLayout", {
width: 170,
height: 80,
textAlignment: "MIDCENTER",
backgroundColor: "#2a80b9",
flexProps: {
flexShrink: 1,
},
});
this.myFlexLayout.addChild(this.label2, "label2", ".sf-flexLayout", {
width: 170,
height: 80,
textAlignment: "MIDCENTER",
backgroundColor: "#8f44ad",
flexProps: {
flexShrink: 5,
},
});
this.myFlexLayout.addChild(this.label3, "label3", ".sf-flexLayout", {
width: 170,
height: 80,
textAlignment: "MIDCENTER",
backgroundColor: "#16a086",
flexProps: {
flexShrink: 10,
},
});
}
}
Before FlexShrink is set
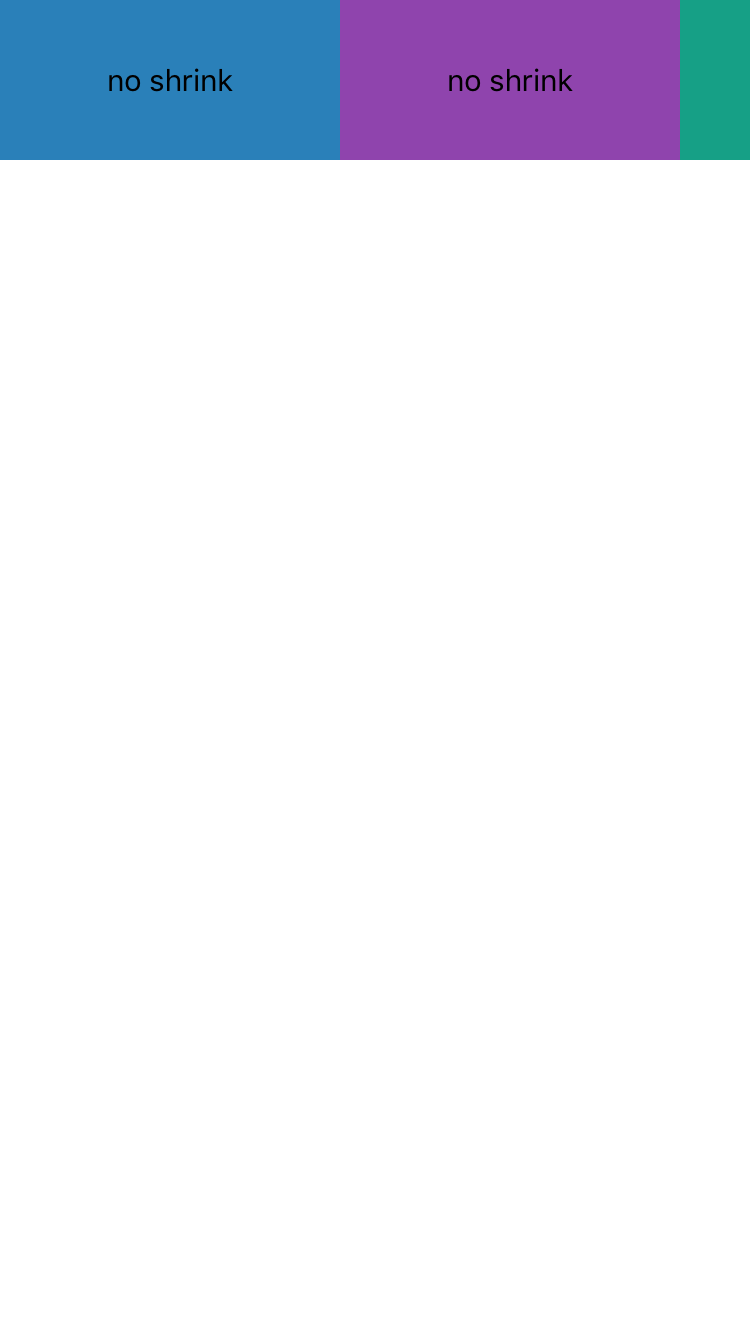
After FlexShrink is set
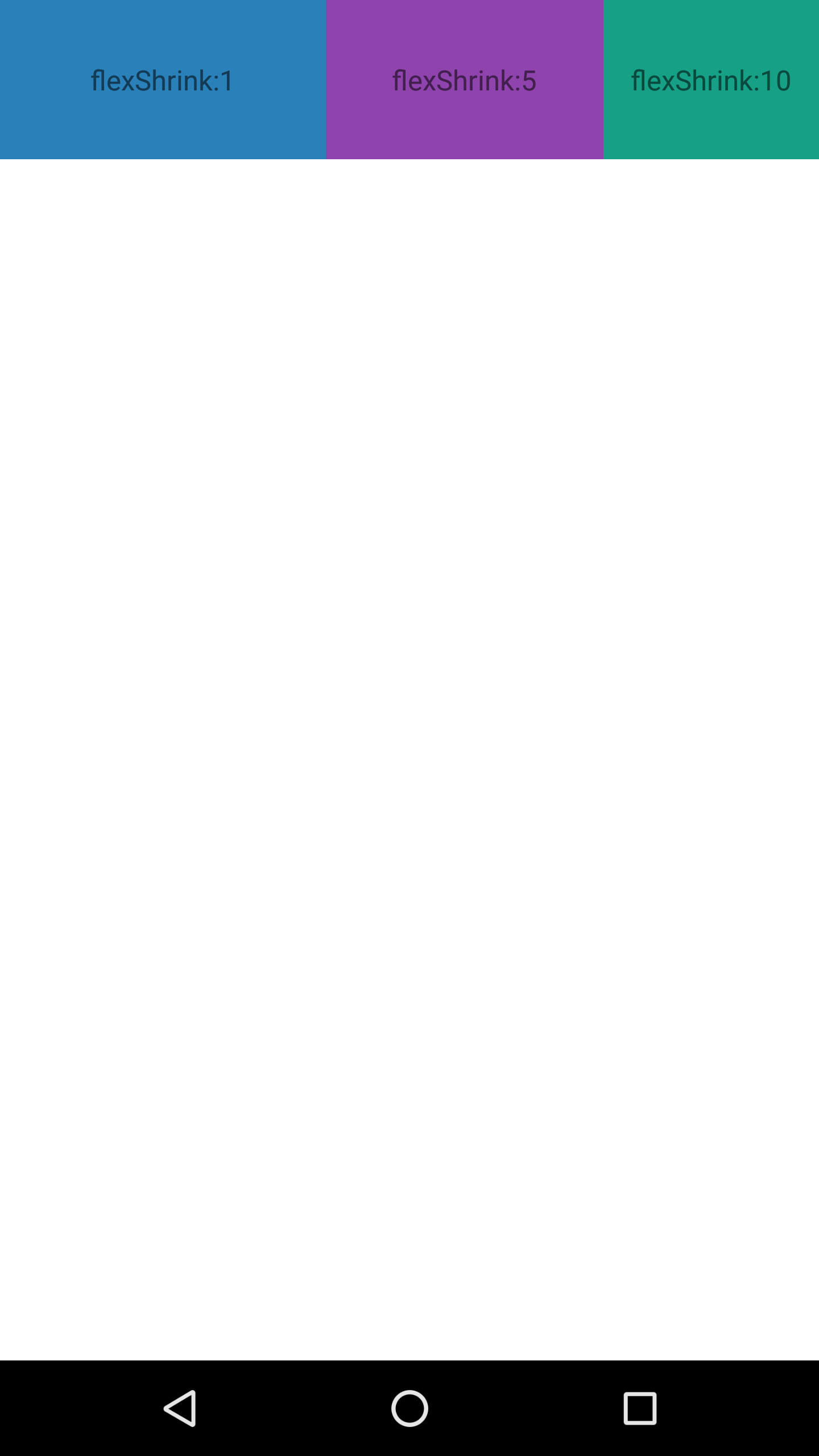