WebView
API Reference: UI.WebView
WebView can show web pages and display custom HTML code. It has basic browser functions such as refresh and page navigation.
Listening URL Changes inside of WebView
Use onChangedUrl callback for invoking any action you desire on Smartce webview.
OnChangedUrl callback triggers when the url is changed. If it returns false, the url cannot rendered inside the webview or on the browser.
openLinkInside property indicates whether the url will be rendered inside the webview or not. If it is set false, the url will be rendered on the browser.
The components in the example are added from the code for better showcase purposes. To learn more about the subject you can refer to:
Adding Component From CodeAs a best practice, Smartface recommends using the WYSIWYG editor in order to add components and styles to your page or library. To learn how to use UI Editor better, please refer to this documentation
UI Editor Basicsimport PageSampleDesign from "generated/pages/pageSample";
import { Route, Router } from "@smartface/router";
import System from "@smartface/native/device/system";
import Application from "@smartface/native/application";
import WebView from "@smartface/native/ui/webview";
import { styleableComponentMixin } from "@smartface/styling-context";
class StyleableWebView extends styleableComponentMixin(WebView) {}
//You should create new Page from UI-Editor and extend with it.
export default class Sample extends PageSampleDesign {
myWebView: StyleableWebView;
constructor(private router?: Router, private route?: Route) {
super({});
}
onShow() {
super.onShow();
const { headerBar } =
System.OS === System.OSType.ANDROID ? this : this.parentController;
Application.statusBar.visible = false;
headerBar.visible = false;
this.myWebView.loadURL("https://www.smartface.io");
}
onLoad() {
super.onLoad();
this.myWebView = new StyleableWebView({
onChangedURL: function (event: any) {
console.log("Event Change URL: " + event.url);
return true;
},
onError: function (event: any) {
console.log("Event Error : " + event.message + ", URL: " + event.url);
},
onLoad: function (event: any) {
console.log("Event Load: " + event.url);
},
onShow: function (event: any) {
console.log("Event Show: " + event.url);
},
});
this.myWebView.android.page = this;
this.addChild(this.myWebView, "myWebView", ".sf-webView", {
left: 10,
top: 10,
right: 10,
bottom: 10,
flexProps: {
positionType: "ABSOLUTE",
},
});
}
}
If you want to upload a image in WebView, webview's page property must be set.
POST requests
HTTP(S) schemes
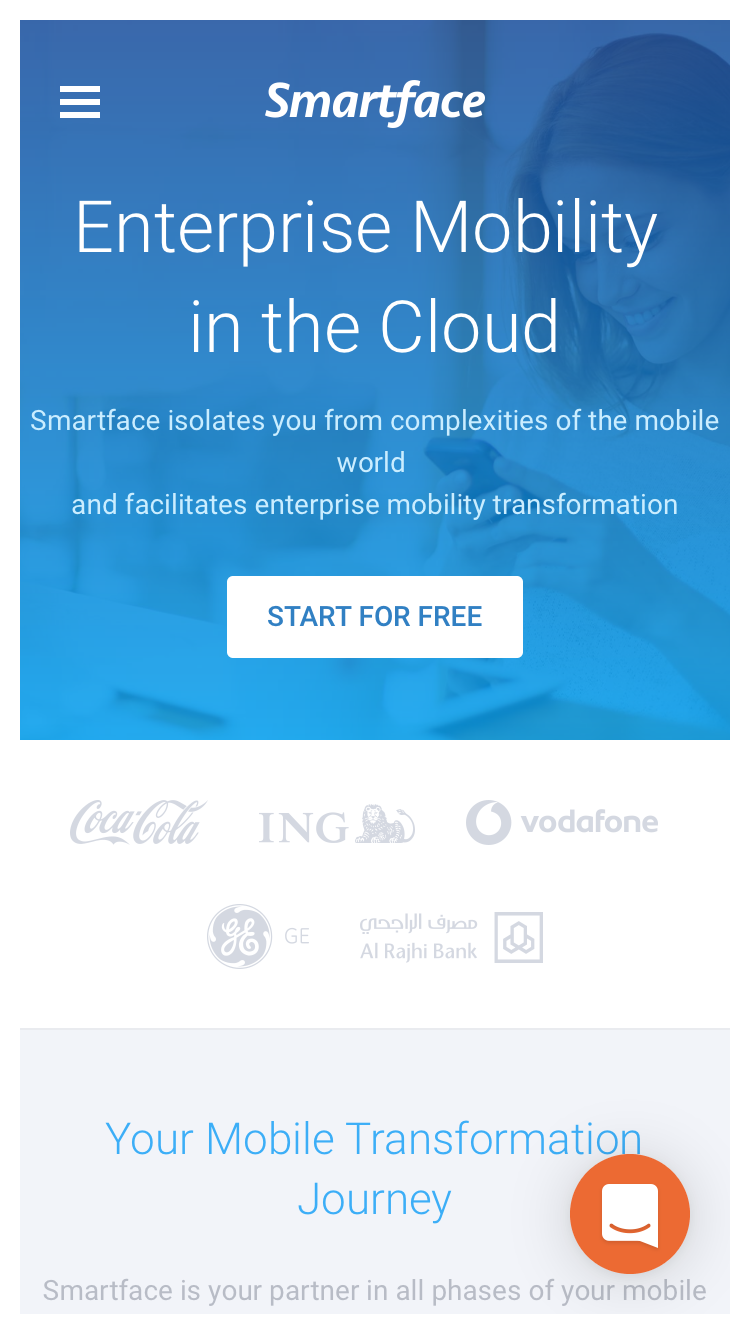
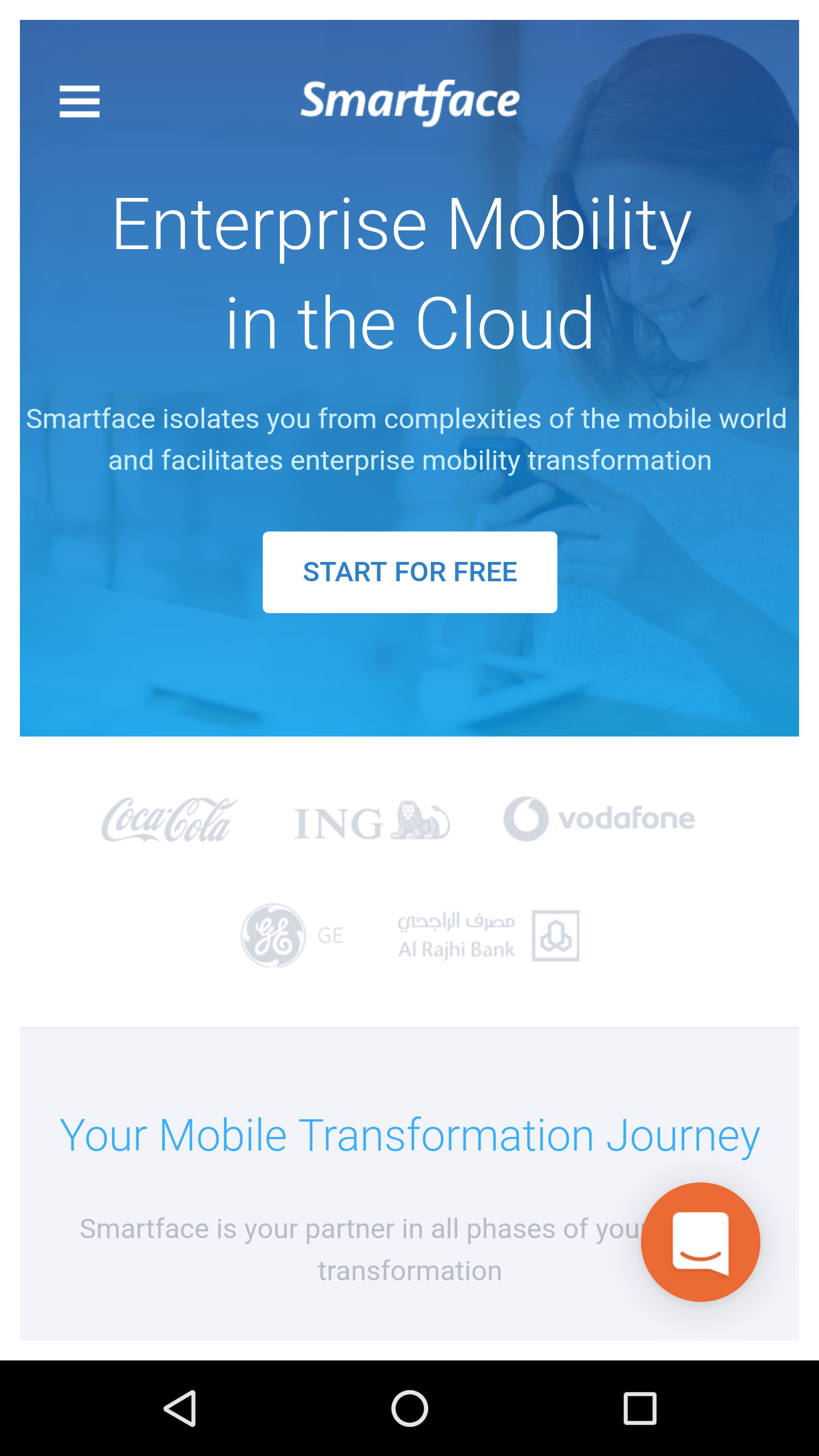
Run JavaScript Script on Webview
Use evaluateJS function for running JavaScript script on Smartface webview. This method takes an callback to handle return value.
A return value that is not inside a function doesn't handle in callback. See the below examples.
let myScript: String = 'return "aaa"';
let callback: Function = function onResult(result) {
console.log("Result " + result);
};
//@ts-ignore
this.myWebView.evaluateJS(myScript, callback); // result is null
let myScript2: String = `
function doSomething() {
return "aaa";
}
doSomething()`;
//@ts-ignore
this.myWebView.evaluateJS(myScript2, callback); // result is "aaa"
Handling User Agent, cookies and custom events
For such cases, Smartface recommends to use webviewbridge utility maintained on Smartface Modules
Webviewbridge allows the developer to define custom user agent and cookie by using customNavigate, ease the usage of web callbacks and injecting bridge codes.